반응형
출처 => C++ How to Program (Detitel / Prenticehall) / 현재 그리고 미래지향적인 C++ 프로그래밍(장석우, 임정목 / 비앤씨에듀케이션)
프랜드 함수란 무엇인가?
▶ C++에서는 크게 세 가지의 접근 지정자가 있다. 모든 곳에서 사용이 가능한 public, 클래스 내부와 상속받은 클래스에서 사용 가능한 protected, 그리고 선언된 클래스 내부에서만 선언이 가능한 private.
▶ 프랜드 함수는 다른 클래스에서는 사용할 수 없는 private 형의 멤버를 사용할 수 있도록 해주는 함수이다.
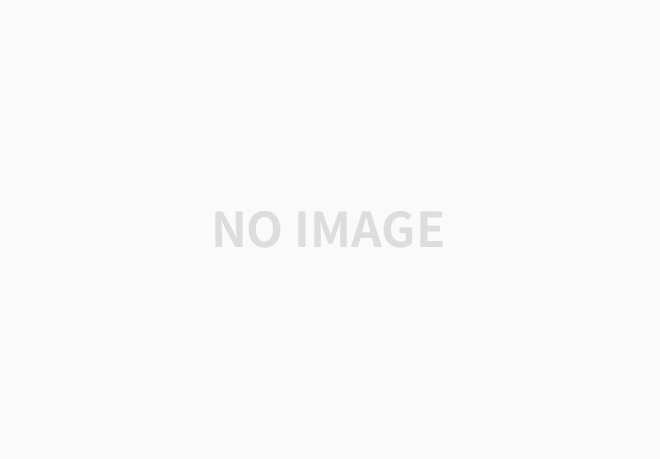
▶ 프랜드 함수로 초대되는 3가지 유형이 존재한다.
1. 클래스 외부에 선언된 전역함수
2. 다른 클래스의 특정 멤버 함수
3. 다른 클래스의 모든 멤버 함수
1. 클래스 외부에 선언된 전역함수 예제
#include<iostream>
using namespace std;
class Rect;
//서로 다른 곳에서 선언이 된 Rect클래스와 equals메소드
class Rect {
int width, height;
public:
Rect(int width, int height) {
this->width = width;
this->height = height;
}
//Rect클래스의 생성자 생성
friend bool equals(Rect r, Rect s);
//전역 함수인 equals함수를 프랜드함수로 불러옴
};
bool equals(Rect r, Rect s) {
if (r.width == s.width && r.height == s.height)
return true;
else return false;
}
void main() {
Rect a(3, 4);
Rect b(3, 4);
if (equals(a, b))
cout << "equal" << endl;
else cout << "not equal" << endl;
}
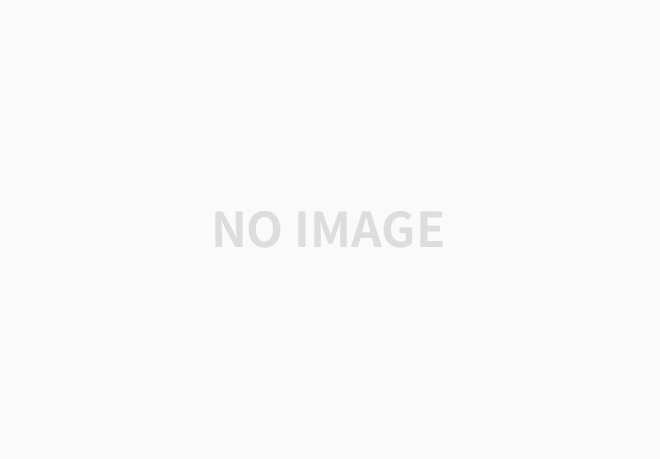
2. 다른 클래스의 멤버함수를 프랜드로 선언하는 예제
#include<iostream>
using namespace std;
class Rect;
class RectManager {
public:
bool equals(Rect r, Rect s);
};
class Rect {
int width, height;
public:
Rect(int width, int height) {
this->width = width;
this->height = height;
}
friend bool RectManager::equals(Rect r, Rect s);
};
bool RectManager::equals(Rect r, Rect s) {
if (r.width == s.width && r.height == s.height)
return true;
else return false;
}
void main() {
Rect a(3, 4);
Rect b(3, 4);
RectManager man;
if (man.equals(a, b))
cout << "equals" << endl;
else cout << "not equals" << endl;
}
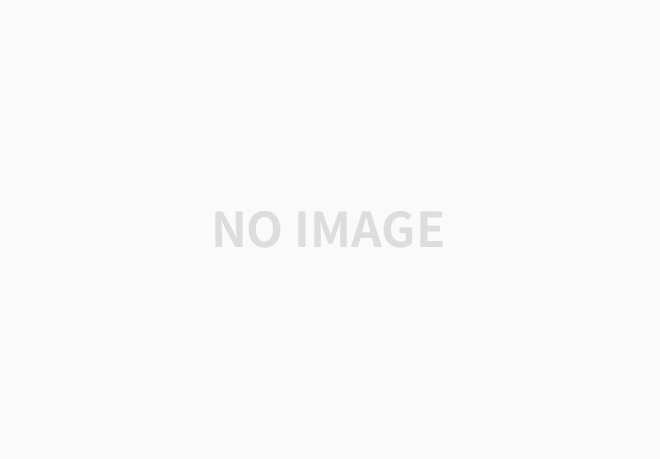
3. 다른 클래스 전체를 프랜드로 선언하는 예제
#include<iostream>
using namespace std;
class Rect;
class RectManager {
public:
bool equals(Rect r, Rect s);
void copy(Rect& dest, Rect& src);
};
class Rect {
int width, height;
public:
Rect(int width, int height) {
this->width = width;
this->height = height;
}
friend RectManager; //RectManager라는 클래스를 프랜드로 선언
};
bool RectManager::equals(Rect r, Rect s) {
if (r.width == s.width && r.height == s.height)
return true;
else return false;
}
void RectManager::copy(Rect& dest, Rect& src) {
//src를 dest에 복사
dest.width == src.width;
dest.height == src.height;
}
void main() {
Rect a(3, 4);
Rect b(3, 4);
RectManager man;
man.copy(b, a);
//b의 멤버변수 값이 a와 같아진다. a를 b에 복사
if (man.equals(a, b))
cout << "equals" << endl;
else cout << "not equals" << endl;
}
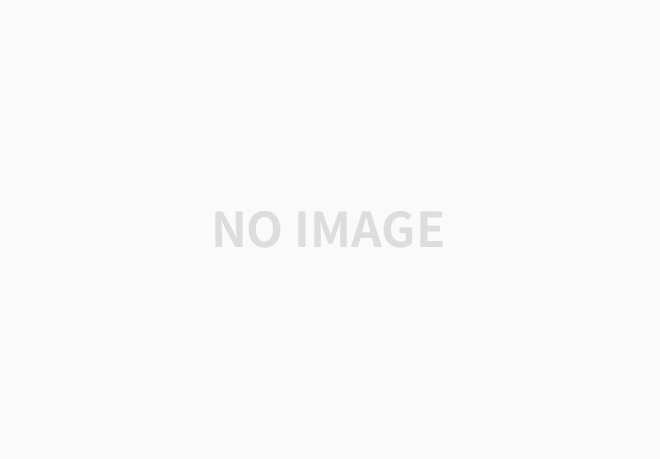
728x90
반응형
'🖥️ 컴퓨터공학 🖥️ > C | C++' 카테고리의 다른 글
[C++] 상속(Inheritance) - 1 (feat. 개념, 상속 접근 지정자) (0) | 2020.06.19 |
---|---|
[C++] 연산자 중복 (feat. 프랜드 함수) (0) | 2020.06.19 |
[C++] 생성자와 소멸자 (0) | 2020.06.18 |
[C++] 포인터 변수 (feat. * / & / **) (0) | 2020.06.17 |
[C++] 조건문 (feat. if 문과 switch 문) (0) | 2020.06.17 |