문제 출처입니다.
https://programmers.co.kr/learn/courses/30/lessons/42588
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
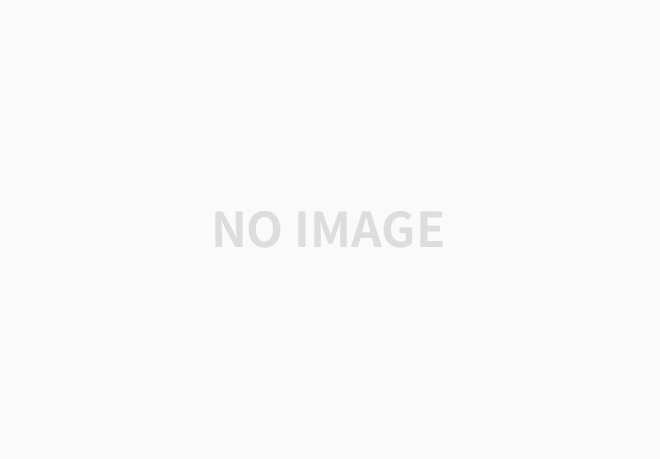
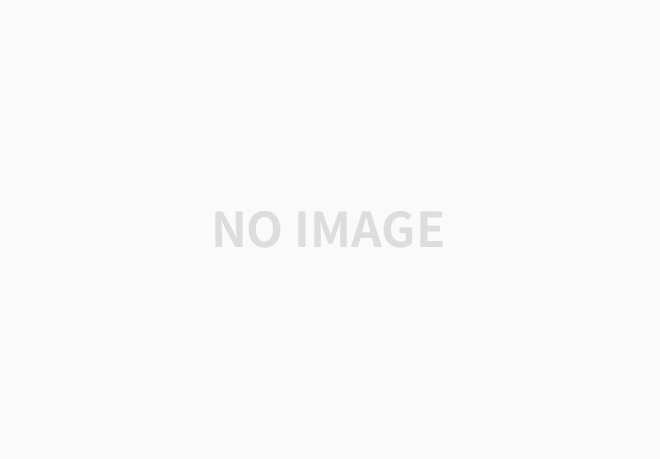
스택/큐 영역에 있어서 스택으로 풀어야 할 것 같았지만 보자마자 배열이 생각나서 배열로 풀어보기로 했다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
package Programmers;
class Solution {
public int[] solution(int[] heights) {
int[] answer = new int[heights.length];
for(int i=heights.length;i>0;i--){
for(int j=1;j<=i;j++){
if(heights[i-j]>=heights[i]){
answer[i]=i-j;
break;
}
if(i==j){
answer[i]=0;
}
}
}
return answer;
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
뭐 이런식으로 작성을 했지만 계속 오류가 나서 다른사람들의 풀이를 보았다..ㅠㅠ
.
.
(↓수정본. 200402)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
class Solution {
public int[] solution(int[] heights) {
int[] answer = new int[heights.length];
for(int i=heights.length-1;i>0;i--){
for(int j=1;j<=i;j++){
if(heights[i-j]>heights[i]){
answer[i]=i-j+1;
break;
}
if(i==j){
answer[i]=0;
}
}
}
return answer;
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
(수정된 코드/ 200402)
결국 풀었다. 풀이 방향은 맞았는데 크게 3가지의 문제점이 있었다.
1. 배열은 0부터 시작. 그러므로 가장 오른쪽 끝에 있는 배열의 인덱스는 heigths.length-1이여야 한다.
2. 신호는 무조건 자신의 탑보다 큰 탑에서 수신이 가능하다. 같으면 수신이 불가능이다.
3. 배열은 0부터 시작이지만 문제에서는 맨 처음 탑의 인덱스를 1로 설정해 주었기 때문에 배열의 인덱스에 +1을 해주어야 한다!
계속 안풀렸는데 오늘 풀렸다! 기분 겁나 좋다!!!!
먼저 배열로 푼 사례
1. 배열
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
class Solution {
public int[] solution(int[] heights) {
int[] answer = new int[heights.length]; // @1
for(int i=heights.length-1; i>=0; i--) { // @2
for(int j=i-1; j>=0; j--) { // @3
if(heights[j] > heights[i]) { // @4
answer[i] = j+1;
break;
}
if(j==0) answer[i] = 0; // @5
}
}
return answer;
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
이 사례 역시 '각각의 탑을 배열로 설정하고 왼쪽으로 송신을 하기 때문에 가장 오른쪽에 있는 탑을 기준으로 왼쪽에 있는 탑과 비교한다'는 점에서 비슷하지만, 중간에 for문에서 j를 어떤 식으로 사용하였는지가 다르다. 여튼 스택을 이용한 풀이보다 훨씬 간단해서 보기 좋은 것 같다.
2. 스택
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
class Solution {
class Tower {
int idx;
int height;
public Tower(int idx, int height) {
this.idx = idx;
this.height = height;
}
//탑에 대한 정보를 담는다
@Override
public String toString() {
return "idx : " + idx + " height : " + height;
}
}
public int[] solution(int[] heights) {
Stack<Tower> st = new Stack<>();
int[] answer = new int[heights.length];
for (int i = 0; i < heights.length; i++) {
Tower tower = new Tower(i + 1, heights[i]);
int receiveIdx = 0;
while (!st.isEmpty()) {
Tower top = st.peek();
receiveIdx = top.idx;
break;
}
}
answer[i] = receiveIdx;
}
return answer;
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
이 풀이가 이 문제의 적합한 정답이라고 한다.
느낀점: 그래도 저번보단 많이 늘은 것 같다. 하지만 너무 부족하다. 하루에 한 단원은 끝내야지.
'💻 개인공부 💻 > 알고리즘' 카테고리의 다른 글
백준) - 1로 만들기(재귀함수) / C++ (0) | 2020.04.13 |
---|---|
백준) - 1로 만들기 (재귀함수) / JAVA (0) | 2020.04.13 |
프로그래머스) - 더 맵게 (우선순위 큐) (0) | 2020.04.04 |
개인) - 단순선택을 이용한 알고리즘 정렬 (0) | 2020.04.04 |
프로그래머스) - 완주하지 못한 선수 (0) | 2020.03.10 |